1
2
3
4
5
6
7
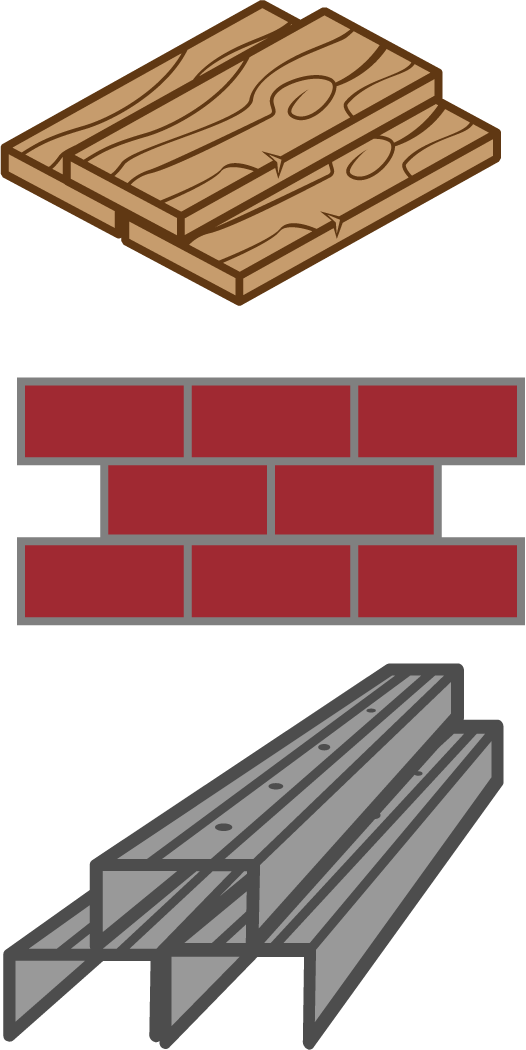
if(contract === 'signed')
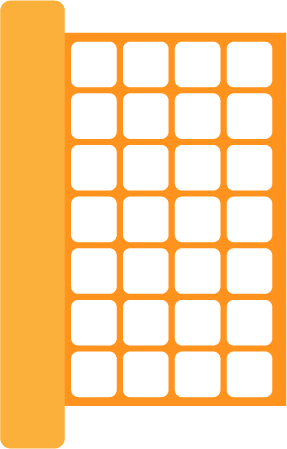
if(siteActive === false) {
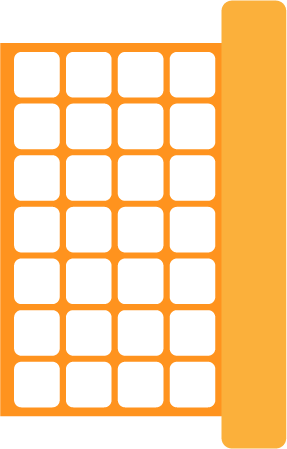
}
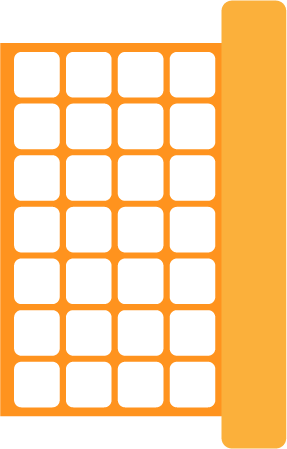
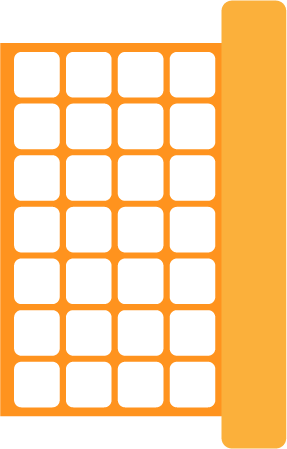
}
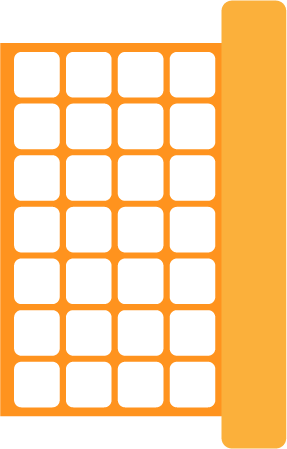
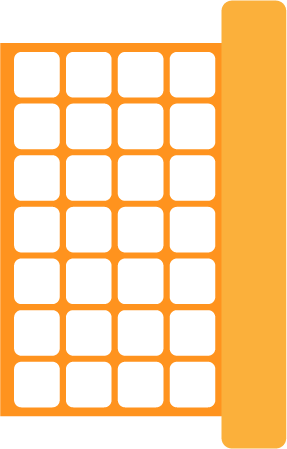
}
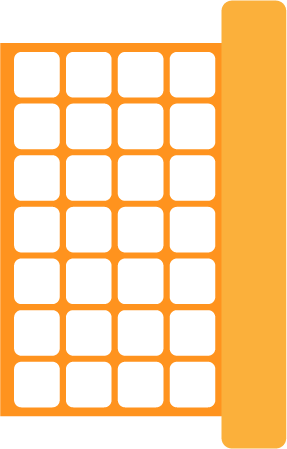
else {
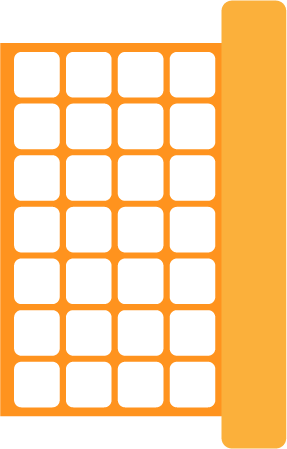
}
archictectureTeam
.technicalWriter
.adminAssistant
.takeNotes()
.holdWeeklyMeeting()
.draftPlans()
crane
.boom
.claw
.pickup()
.drop()
.reverse()
.rotate()
.accelerate()
.decelerate()
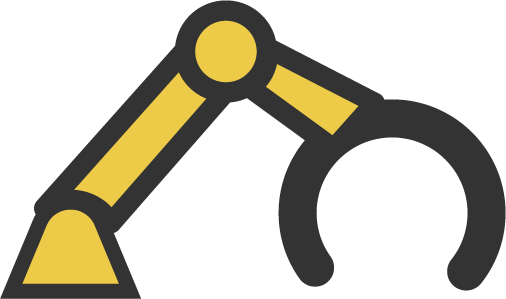
crane.pickup('wood');
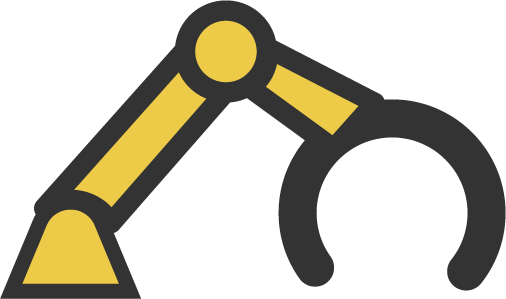
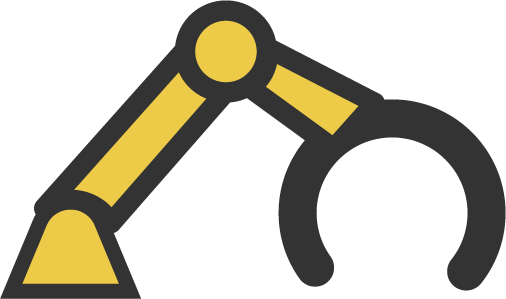
function addBrick(count){
8
return count + 1;
}
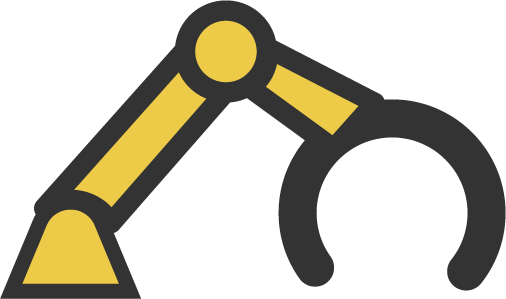
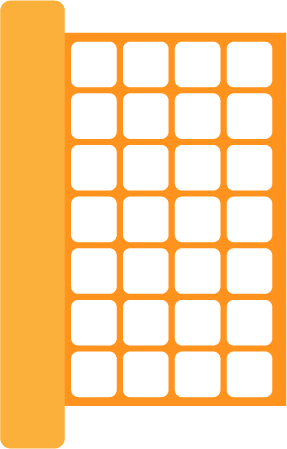
8
function addBrick(count){
8
return count + 1;
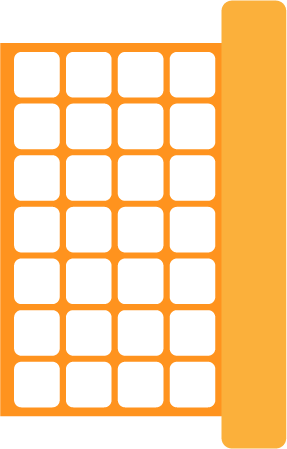
}
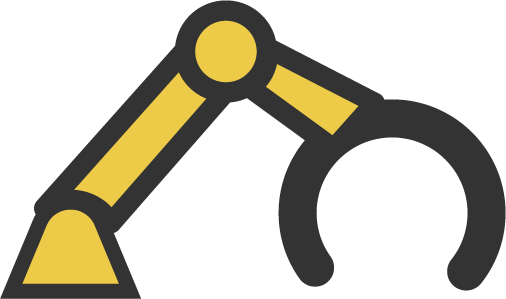
8
addBrick(bricks)
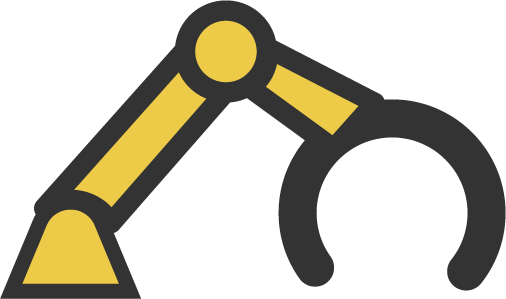
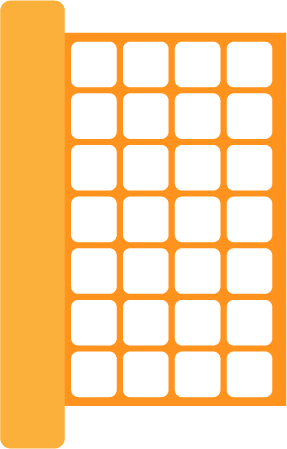
function addBrick(count){
20
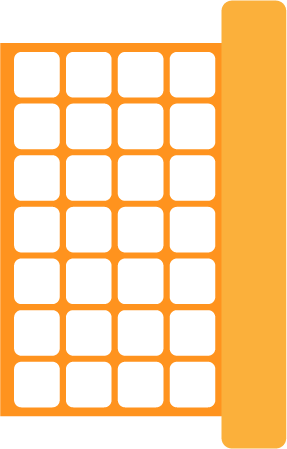
}
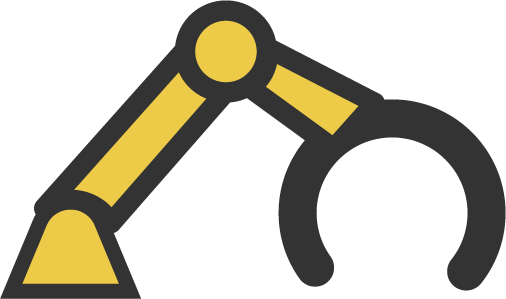
addBrick(bricks)
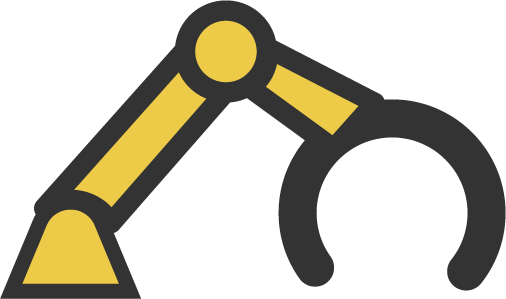
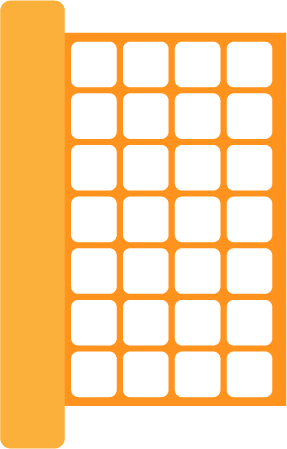
20
function addBrick(count){
20
return count * 2;
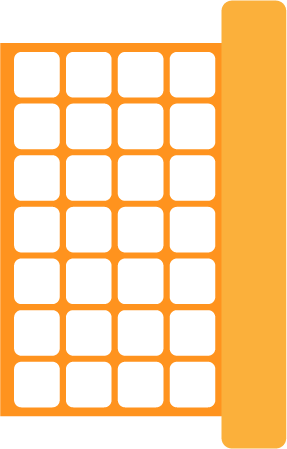
}
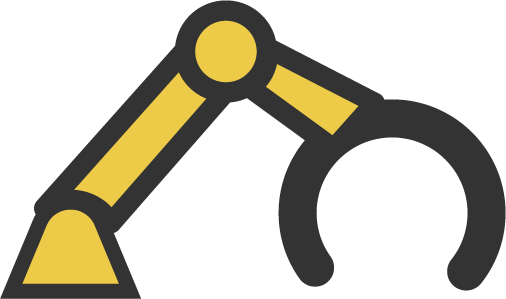
20
var finalBrickCount= addBrick(bricks)
Step 5/5 - We load up the result of the addBrick() function into the finalBrickCount truck.
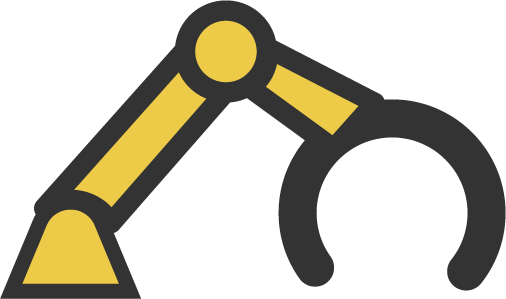
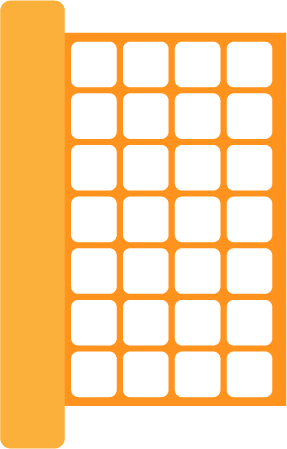
10
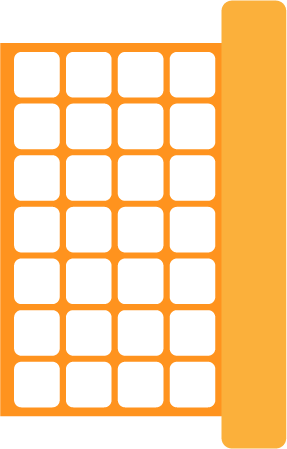
}
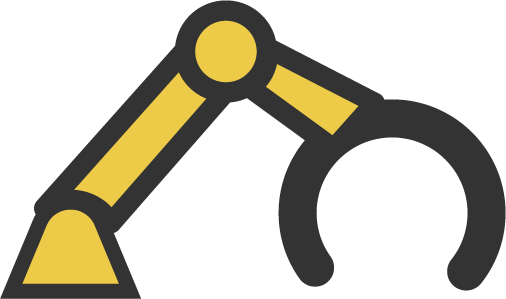