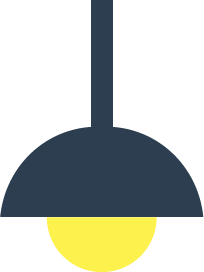
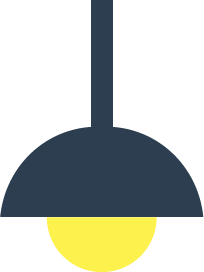
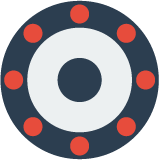
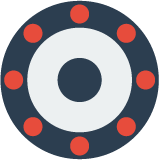
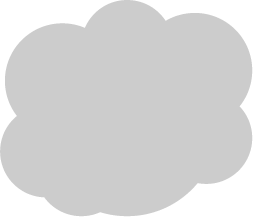
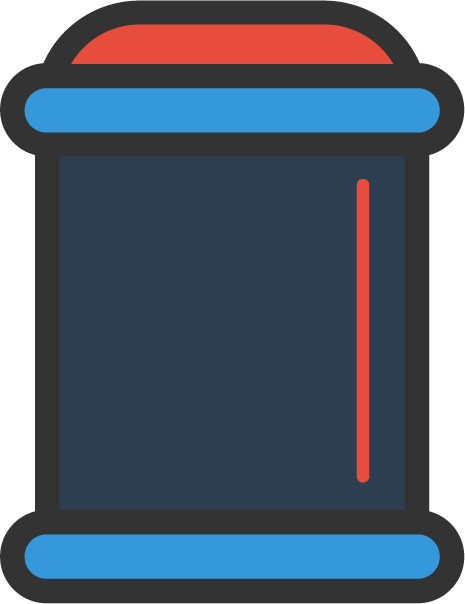
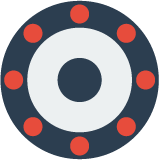
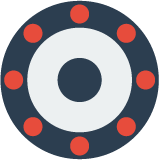
Legs
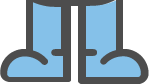
Body
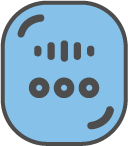
Arms
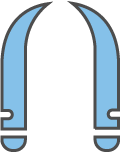
Head
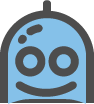
9
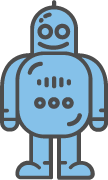
8
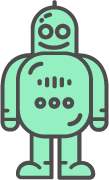
7
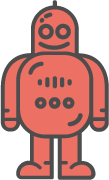
6
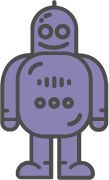
5
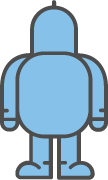
4
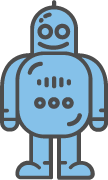
3
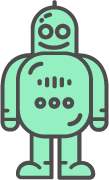
2
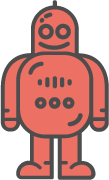
1
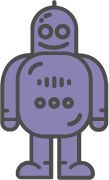
0
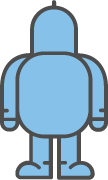
-
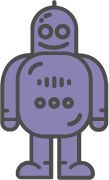
Learn the basics of 'for' loops as well as higher order functions like .map() in Javascript
Legs
Body
Arms
Head
9
8
7
6
5
4
3
2
1
0
-
The basics of iterating through arrays and objects
For Loop Basics, 11 steps
Higher order functions and more advanced techniques
.map() Functions, 10 steps
Learn the basics of 'for' loops and how to use them with arrays and objects
var robots = ['blueRobotBackwards', 'purpleRobot', 'redRobot', 'greenRobot', 'blueRobot', 'blueRobotBackwards', 'purpleRobot', 'redRobot', 'greenRobot', 'blueRobot']
Check out the array above. There are 10 items, which are shown in the window on the left. 'For' loops allow you to iterate through this array. You can take an action with each item in the array in a programatic way, as opposed to selecting them one by one.
All the time.
Docs
How to choose specific items in arrays
var robots = [ 'blueRobotBackwards', 'purpleRobot', 'redRobot', 'greenRobot', 'blueRobot', 'blueRobotBackwards', 'purpleRobot', 'redRobot', 'greenRobot', 'blueRobot' ]
robots[1]= 'purpleRobot'
robots[9]= 'blueRobot'
robots[4]= 'blueRobot'
Arrays are 0-indexed. This means that the first item in the array is in the 0 position. Check out the display on the left!
Select the seventh item in the robots array
Recall that the first item in the array is labeled 0!
How JavaScript does for loops, a fundamental programming construct
var robots = [ 'blueRobotBackwards', 'purpleRobot', 'redRobot', 'greenRobot', 'blueRobot', 'blueRobotBackwards', 'purpleRobot', 'redRobot', 'greenRobot', 'blueRobot' ]
for(var i=0; i < robots.length; i++){}
var i=0;= Create a variable called 'i' that starts at 0
i < robots.length;= i must always be less than 10, the length of the array
i++= Increase i by 1 every time the loop finishes, and restart it
Set 0 as the start point with a variable called i
Make sure i is limited by the length of robots array
Increase i by 1 after every iteration
Docs
Do your answers match those in the example?
Once we are in the 'for' loop, we can do anything to each item!
Alright, running the loop in the previous step was a little misleading. We need to do something to each item in the array! In this case, we will create a new array for the second conveyor belt, and add items to that.
var belt2 = [];
for(var i = 0; i < robots.length; i++){ belt2.push(robots[i])
}
var belt2 = [];= Create a variable called 'belt2' that is an empty array.
.push()= Add each item to the end of the belt2 array.
robots[i]= Choose the item based on i, the variable that you increment in the for loop statement
Create an empty array called 'conveyor2'
Add each item from the robots array using the .push() method
Docs
Are you picking each item in the array correctly?
Let's dig deeper into each part of the for loop
i is normally initialized to 0, but what happens if we start in the middle of the array?
var belt2 = [];
for(var i = 5; i < robots.length; i++){ belt2.push(robots[i])
}
var i = 5;= Start from the item with an index of 5, the 6th item in the array.
See how the first 5 items never get touched? That is because you started at index 5, and only go up from there.
Make i start with the 3rd item in the array
Docs
Remember, the count starts at 0, so the 3rd item has index 2!
Let's dig deeper into each part of the 'for' loop
If you want to run through every item in the array, you usually set the upper bound to the full length of the array. But what if you do not want to use every item?
var belt2 = [];
for(var i = 0; i < 4; i++){ belt2.push(robots[i])
}
i < 4= Stop the for loop after iterating through the first 4 items
See how the last 6 items never get touched? That is because you end before index 4
Only iterate through the first two items of the array
Docs
You only want to iterate through the elements in positions 0 and 1
Let's dig deeper into each part of the 'for' loop
The previous examples assume that you want to iterate through each item. But what if you only want to iterate through items with an odd or even index?
var belt2 = [];
for(var i=0; i < robots.length; i+=2){ belt2.push(robots[i])
}
i+=2= Iterate through every other item, beginning with the 0 index. i +=2 is the same as i = i+2.
See how the odd-indexed items never get touched? i is never odd (in this case)
Only iterate through every third item
Docs
You need to add 3 to i with every iteration of the loop
Let's learn more about the object data structure
var robots = [ {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'}, {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'} ]
var belt2 = [];
for(var i=0; i < robots.length; i++){ if(robots[i]['color'] == 'blue'){
belt2.push(robots[i]) }
}
robots[i]['color']= Gets the color property of each object in the robots array
== 'blue'= If the string from the object is exactly 'blue'
Write condition that stipulates that you will only push items if their color is 'purple'
Docs
Check out the example above to see how to choose based on a color
Let's learn more about the object data structure
var robots = [ {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'}, {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'} ]
var belt2 = [];
for(var i=0; i < robots.length; i++){ if(robots[i]['color'] == 'blue'{
belt2.push(robots[i]) }
}
Write condition that stipulates that you will only push items if their orient is 'back'
Docs
Check out the example above to see how to choose based on a color
The forEach() method executes a provided function once per array element.
var robots = [ {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'}, {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'} ]
var belt2 = [];
robots.forEach(function(element, index) {
belt2.push(element)
})
forEach offers another way to handle for loops. Notice that you do not need to explicitly use an index with the variable i to outline each element. The element argument covers that functionality.
.forEach()= Iterates through each item in the array. No need to create a variable i! But less flexibility.
function(element, index)= Each element in the array can be called explicitly. The index argument allows you to choose elements based on their index.
Push the entire robots array to belt2
Docs
Make sure that you used function with both arguments!
The forEach() method executes a provided function once per array element.
var belt2 = [];
robots.forEach(function(element, index) {
if(element.color == 'green'){ belt2.push(element)
}
})
element.color= Another way to access the property of each object in the array. Same as element['color']
Only return red robots
Docs
Make sure that you call the property correctly!
Learn to write cleaner code with higher order functions
var robots = [ 'blueRobotBackwards', 'purpleRobot', 'redRobot', 'greenRobot', 'blueRobot', 'blueRobotBackwards', 'purpleRobot', 'redRobot', 'greenRobot', 'blueRobot' ]
var belt2 = [];
for(var i = 0; i < robots.length; i++){ belt2.push(robots[i])
}
var belt2 = robots.map(function(element,index){
return element
})
Check out the two ways to iterate above. The .map() method is a higher-order function. The .map() method is also an abstraction . It covers the same functionality as a normal 'for' loop, but in a much more succinct way. In fact, .map() is an example of declarative programming.
Docs
The ways it beats a 'for' loop
1. Less lines of code = less bug opportunities
2. Easier to read
3. Can chain multiple calls together
4. Works on data that is available asynchronously i.e. not all at once
Learn to write cleaner code with higher order functions
var robots = [ 'blueRobotBackwards', 'purpleRobot', 'redRobot', 'greenRobot', 'blueRobot', 'blueRobotBackwards', 'purpleRobot', 'redRobot', 'greenRobot', 'blueRobot' ]
var belt2 = robots.map(function(element,index){
return element
})
function(element,index)= A callback with two arguments: element, or each element in the array, and index, or the index of that particular element
return element= For each element in the array, we return the element itself. In other words, the belt2 array will be the same elements as robots.
Start your map() statement by creating a variable 'conveyor2'
Map the array 'robots' to an element and index for each element in the array
Return each element
Docs
Does your statement follow the same structure as the example?
Learn to write cleaner code with higher order functions
var robots = [ {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'}, {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'} ]
Now we are using an array of objects. We can use .map() to return robots with a specific property.
var belt2 = robots.map(function(element,index){
if(element.color == 'blue')
return element
})
if(element.color == 'blue')= As we iterate through each element, check if its color property matches 'blue'. If it does, add that element to belt2.
Start your map() statement by creating a variable 'array2'
Map the array 'robots' to an element and index for each element in the array
Return each element that has a color 'green'
Docs
Does your statement only return elements with the color 'green'?
Turn an array into a useful object
var robotParts= [ ['head.png', 'head'], ['arms.png', 'arms'], ['body.png', 'body'], ['legs.png', 'legs'] ]
Check out the array above. It contains a series of arrays- the first item in each array is the image path, the second item is the location on the body that the part should be mapped to. How might we better organize this?
var robotBody= [{head: 'head.png'},
{arms: 'arms.png'},
{body: 'body.png'},
{legs: 'legs.png'},
}]
We basically need to turn the array inside out. It is currently unorganized, and we need to organize by body section in an object.
Remember that we must return something on every iteration from the original array. In this case we will return an object.
var onePart = {}
Each part has a key-value pair. The key is the second item in the array. The value is the first item.
onePart[key] = value;
Docs
Turn an array into a useful object
var robotParts= [ ['head.png', 'head'], ['arms.png', 'arms'], ['body.png', 'body'], ['legs.png', 'legs'] ]
Needs to become...
var robotBody= [{head: 'head.png'},
{arms: 'arms.png'},
{body: 'body.png'},
{legs: 'legs.png'},
}]
Start your map() statement by creating a variable 'robotBody'
Map the array 'robotParts' to an element and index for each element in the array
Create an empty object called 'onePart' in each iteration
Docs
Does your statement create an empty object called 'onePart' in each case?
Turn an array into a useful object
var robotParts= [ ['head.png', 'head'], ['arms.png', 'arms'], ['body.png', 'body'], ['legs.png', 'legs'] ]
Needs to become...
var robotBody= [{head: 'head.png'},
{arms: 'arms.png'},
{body: 'body.png'},
{legs: 'legs.png'},
}]
var robotBody = robotParts.map(function(element,index){
var onePart={}
})
Each onePart object should have a key with the second item from each array, and a value with the first item of each array
Return the onePart object on each iteration
Docs
Did you map the items from each array to a key and value?
Higher order functions allow for more advanced functionality in fewer lines.
var robots = [ {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'}, {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'} ]
Check out the array above. What if you want to only return the green robots? Sure, you could use an 'if' statement within a map function. That would not be the functional programming approach. Functional programming focuses on making code more readable for any viewer. Although this example will be small, it becomes a much bigger deal with a massive code base that many people use.
var greenBots= [...]
This is what we want to end with.
We need to use a .filter() function to first sort out the green robots. Then we will add the results to the greenRobots array.
var greenBots = robots.filter().map();
We can chain a map method to our filter method.
Docs
The .filter() method
var robots = [ {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'}, {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'} ]
We can use .filter() to return robots with a specific property.
var greenBots = robots.filter(function(element,index){
return element.color =='red'
})
This could be an 'if' statement within a .map(). But, when you have many instances of this within your code base, it becomes harder for others to read your code. It is easier to separate this statement.
Start your filter() statement by creating a variable 'greenBots'
Filter the array 'robots' so you only return green robots
Docs
Does your statement only return elements with the color 'green'?
Putting it all together
var robots = [ {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'}, {color:'blue' , orient:'back'}, { color:'purple' , orient:'front'}, { color:'red' , orient:'front'}, {color:'green' , orient:'front'}, { color:'blue' , orient:'front'} ]
We can use .filter() to return robots with a specific property.
var greenBots = robots.filter(function(element,index){
return element.color =='green'
}).map(function(element, index){
})
The .filter() method results in 2 green robots. Now we need to move those robots to the second belt.
Write the return statement within the .map() function. You want to return every element's color since you already filtered the list down to just green elements.
Docs
Does your statement only return all element colors?
Now go build your own project!